Table Of Content

Design patterns provide a standard terminology and are fundamental to efficient communication among developers. They allow developers to discuss possible solutions efficiently, as they provide a common language for certain complex structures. Moreover, design patterns encourage code reuse, reducing the overall development effort. Java design patterns are divided into three categories - creational, structural, and behavioral design patterns.
The Relevance of Design Patterns in Large-Scale Software Development
This pattern is used when we want to provide controlled access to functionality. This article serves as an index for all the Java design pattern articles. Javatpoint provides tutorials with examples, code snippets, and practical insights, making it suitable for both beginners and experienced developers. Lets you save and restore the previous state of an object without revealing the details of its implementation. Turns a request into a stand-alone object that contains all information about the request.
Creational Software Design Patterns in Java
It's useful when you have multiple ways to solve a problem and want to choose an algorithm at runtime. The Singleton Pattern restricts the instantiation of a Class and ensures that only one instance of the class exists in the Java Virtual Machine (JVM). The implementation of the Singleton Pattern has always been a controversial topic among developers. The sticking point is that singletons remain in memory for the life of an application, making them a potential drain on system resources. On the other hand, having one object in memory is preferable to having many instances existing simultaneously.
Diving Deeper into React JS: Exploring Advanced Concepts
We also have a Context class that uses the State interface to change its behavior based on its current state. The Decorator pattern is useful for adding and removing responsibilities from objects at runtime. It provides a flexible alternative to subclassing for extending functionality. However, it can result in many small objects that can be hard to track and debug.
Software creational patterns can help us when we want to create objects in some certain scenarios, which increase flexibility. The Observer pattern is a design pattern where an object (known as a subject) maintains a list of objects depending on it (observers), automatically notifying them of any changes to state. The Singleton pattern is a design pattern that restricts the instantiation of a class to a single instance. This is useful when exactly one object is needed to coordinate actions across the system. The Iterator pattern provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation. It's useful when you want to traverse a data structure without knowing its implementation details.
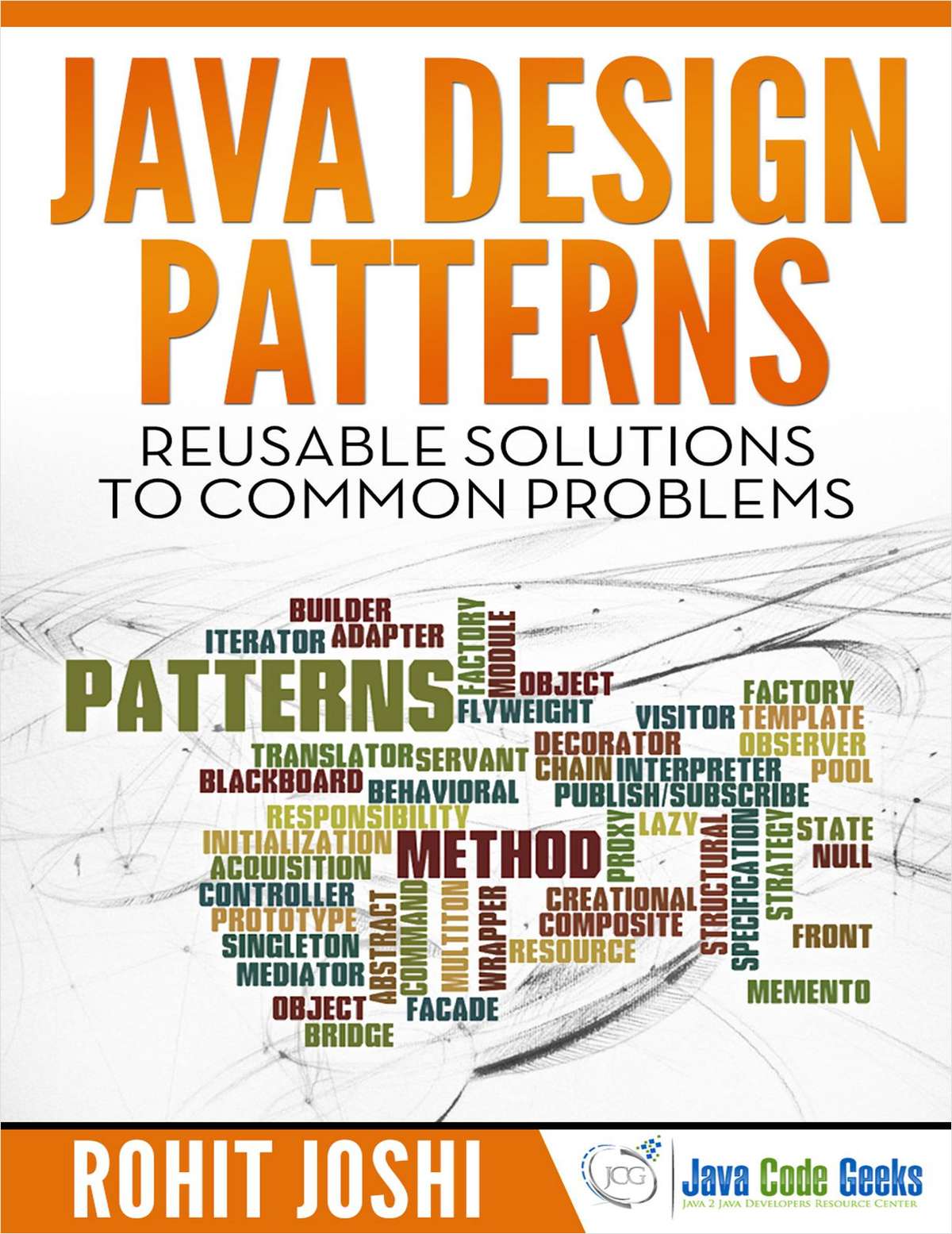
We also touched on common issues that developers may encounter when implementing design patterns, such as misuse and overuse, and provided solutions and workarounds for these issues. In the context of software development, a design pattern is a reusable solution that can be applied to common problems encountered in software design. They represent best practices evolved over a period of time and are named in a way that can convey their intent. When implementing design patterns in Java, developers often run into a few common issues. Let’s explore these problems and provide some solutions and workarounds. They provide reusable solutions to common problems, allowing you to write cleaner, more maintainable code.
Memento pattern is implemented with two Objects – originator and caretaker. The originator is the Object whose state needs to be saved and restored, and it uses an inner class to save the state of Object. The inner class is called “Memento”, and it’s private so that it can’t be accessed from other objects. We know that we can have multiple catch blocks in a try-catch block code. Here every catch block is kind of a processor to process that particular exception.
It's useful when you want to maintain consistency between related objects. The Decorator pattern allows you to attach additional responsibilities to an object dynamically. The Composite pattern allows you to compose objects into tree structures to represent part-whole hierarchies. It enables clients to treat individual objects and compositions uniformly.
The Role of Design Patterns in Java Programming
Source code and design conformance, design pattern detection from source code by classification approach - ScienceDirect.com
Source code and design conformance, design pattern detection from source code by classification approach.
Posted: Fri, 11 Oct 2019 05:39:09 GMT [source]
AccountType consists of fixed values that have been predefined in the banking industry domain; therefore, it is a constant, and we use Enum for this data type to ensure integrity. The end result is retrieved from the builder object because the director can’t know the type of resulting product. In this example, the Builder pattern allows step by step construction of different car models. If we do not use the state pattern in such cases, we will end up having lots of ”if-else” statements which make the code base ugly, unnecessarily complex, and hard to maintain. So the things that come from the user interface, translate into a command interface which has an “execute” method and the process goes on.
Momento Method is a Behavioral Design Pattern, it provide to save and restore the previous state of an object without revealing the details of its implementation. This establishes a central store for the theme value (initially set to ‘light’). The App component wraps its children (Toolbar and Content) with a ThemeContext.Provider, making the current theme value available to them and any nested components. Both Toolbar and Content can then access the theme using useContext(ThemeContext). First, we create a BankAccount, which is an abstract class that will contain information commonly used for specific Accounts. It is useful when you need to implement undo/redo functionality or need to save and restore the state of an object without exposing its internal details.
Each handler decides whether to process the request or pass it to the next handler in the chain. The Proxy pattern provides a surrogate or placeholder for another object to control access to it. It's useful when you want to add a layer of indirection between the client and the real object. The Flyweight pattern uses sharing to support large numbers of fine-grained objects efficiently. It's useful when you have a large number of objects that share a common state and can be replaced by a single shared object. The Adapter pattern allows you to convert the interface of a class into another interface that clients expect.
There are different groups of design patterns in software design which we only talked about “Creational patterns”. Sometimes, developers force the use of design patterns where they’re not needed, leading to unnecessary complexity. The Factory pattern provides a way to encapsulate a group of individual factories that have a common theme without specifying their concrete classes.
No comments:
Post a Comment